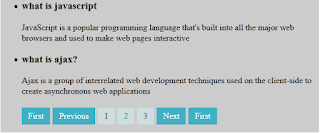
Below are step by step guide so that you can easly add this pagination in your site.
Step 1
create a database and add the following table along with data dump.CREATE TABLE IF NOT EXISTS `articles` ( `id` int(11) unsigned NOT NULL AUTO_INCREMENT, `title` varchar(100) NOT NULL, `date` date NOT NULL, `description` text NOT NULL, `status` tinyint(4) NOT NULL DEFAULT '1', PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8 AUTO_INCREMENT=1 ; INSERT INTO `articles` (`id`, `title`, `date`, `description`, `status`) VALUES (1, 'What is php?', '2013-06-08', 'PHP is a server-side scripting language designed for web development but also used as a general-purpose programming language.', 1), (2, 'What is ASP?', '2013-06-08', 'An ASP file can contain text, HTML tags and scripts. Scripts in an ASP file are executed on the server. What you should already know', 1), (3, 'what is javascript', '2013-06-08', 'JavaScript is a popular programming language that''s built into all the major web browsers and used to make web pages interactive', 1), (4, 'what is ajax?', '2013-06-08', 'Ajax is a group of interrelated web development techniques used on the client-side to create asynchronous web applications', 1), (8, 'what is Jquery?', '2013-06-09', 'The purpose of jQuery is to make it much easier to use JavaScript on your website. What You Should Already Know', 1);
Step 2
Add the Jquery library in head section with following java script code.Step 3
Small css to control the listing and give some style to pagination. You can modify or user your own..container{ margin:0px auto; width:600px; background:#ccc; } /* Pagination =========================================================*/ .pagination { margin:25px 0 5px 0; overflow:hidden; margin:0; list-style:none; } .pagination li { margin:5px 5px 15px 0; float:left; } .pagination li a{ padding:6px 12px; color:#595959; display:block;background-color: #CEDDE0; text-decoration:none; cursor:pointer;} .pagination .current{ color:#228f9c; } .pagination li a.prev, .pagination li a.next{ color:#fff; background:#41b1c5; cursor:pointer; } .pagination li a.prev:hover, .pagination li a.next:hover{ background:#228f9c !important; text-decoration:none; cursor:pointer; }
Step 4
Fetch the data from database when the page is load.include("pages.php"); $max_records=2; $page = "Select * from articles where status=1 order by id asc"; $sql = "Select * from articles where status=1 "; $rstotal=mysql_query($sql) ; $total_records=mysql_num_rows($rstotal); $current_page=(isset($_GET["p"]) && intval($_GET["p"])<>0)?intval($_GET["p"])-1:0; $start=($current_page*$max_records); if($start=='') $start=0; $end=0; if($total_records >= 1) { $total_pages=ceil($total_records/$max_records); if($start < $total_records) { if($start + $max_records < $total_records) $end = $start + $max_records; else $end = $total_records; } $page = "$page LIMIT $start,$max_records"; } <div class="container"> <ul class="data"> <?php if($total_records>0){ $page_rs=mysql_query($page); $page_count=mysql_num_rows($page_rs); while($page_row=mysql_fetch_assoc($page_rs)){?> <li> <h3><?php echo $page_row['title'];?></h3> <div class="desc"> <?php echo $page_row['description'];?> </div> </li> <?php } }else {?> <div align="center"> <?php echo 'no data to show';?> </div> <?php } ?> </ul> <?php if($total_records>$max_records){ ?> <ul class="pagination"> <?php echo paginate($current_page,$total_pages); ?> </ul> <?php }?> </div>
Step 5
Shows the data from database when ajax request is send.<?php header("Content-type:application/json"); if(isset($_POST,$_POST['p']) && $_SERVER['REQUEST_METHOD']=='POST'){ include("pages.php"); $max_records=2; $page = "Select * from articles where status=1 order by id asc"; $sql = "Select * from articles where status=1 "; $rstotal=mysql_query($sql) ; $total_records=mysql_num_rows($rstotal); $current_page=(isset($_POST["p"]) && intval($_POST["p"])<>0)?intval($_POST["p"])-1:0; $start=($current_page*$max_records); if($start=='') $start=0; $end=0; if($total_records >= 1) { $total_pages=ceil($total_records/$max_records); if($start < $total_records) { if($start + $max_records < $total_records) $end = $start + $max_records; else $end = $total_records; } $page = "$page LIMIT $start,$max_records"; } $html=''; $pages=''; if($total_records>0){ $page_rs=mysql_query($page); $page_count=mysql_num_rows($page_rs); while($page_row=mysql_fetch_assoc($page_rs)){ $html.='<li> <h3>'. $page_row['title'].'</h3> <div class="desc"> '. $page_row['description'].' </div> </li>'; } if($total_records>0){ $pages.=paginate($current_page,$total_pages); } echo json_encode(array('status'=>true,'html'=>"$html",'pages'=>"$pages"));exit; }else { $html.=' <div align="center"> '. 'no data to show </div>'; echo json_encode(array('status'=>true,'html'=>"$html"));exit; } }else{ echo json_encode(array('status'=>false,'msg'=>"Sorry some unexpected error occur. please try again."));exit; } ?>
Step 6 Final step
pagination function create the file name pages.php and add this script.
function paginate($current = 1, $total) { global $max_records; $current++; $perpage=3;// per page link if(!empty($max_records)) $limit=$max_records; if(!$current) $current = 1; $last = ceil($total); $previous=$current - 1; $next=$current + 1; $pagination=''; if($total > 1) { if( $current >1) { $pagination.="<li ><a rel='1' class='prev pages' >First</a></li>"; $pagination .= "<li ><a rel=".$previous." class='prev pages'>Previous</a></li>"; } else { } if ($current >= $perpage) { $pages = $current - floor($perpage/2); if ($last > $current + floor($perpage/2)) if($perpage%2==0) $loop = $current + floor($perpage/2)-1; else $loop = $current + floor($perpage/2); else if ($current <= $last && $current > $last - ($perpage-1)) { $pages = $last - ($perpage-1); $loop = $last; } else { $loop = $last; } } else { $pages = 1; if ($last > $perpage) $loop = $perpage; else $loop = $last; } for($i = $pages; $i <= $loop; $i++) { $class=($current==$i)?'current':''; $pagination.="<li ><a class='".$class." pages' rel='".$i."'>".$i."</a></li>"; } if( $total != $current ) { $pagination .= "<li ><a rel=".$next." class='prev pages'>Next</a></li>"; $pagination.="<li ><a rel='".$total."' class='prev pages' >Last</a></li>"; } else { } } return $pagination; }
View Demo Download